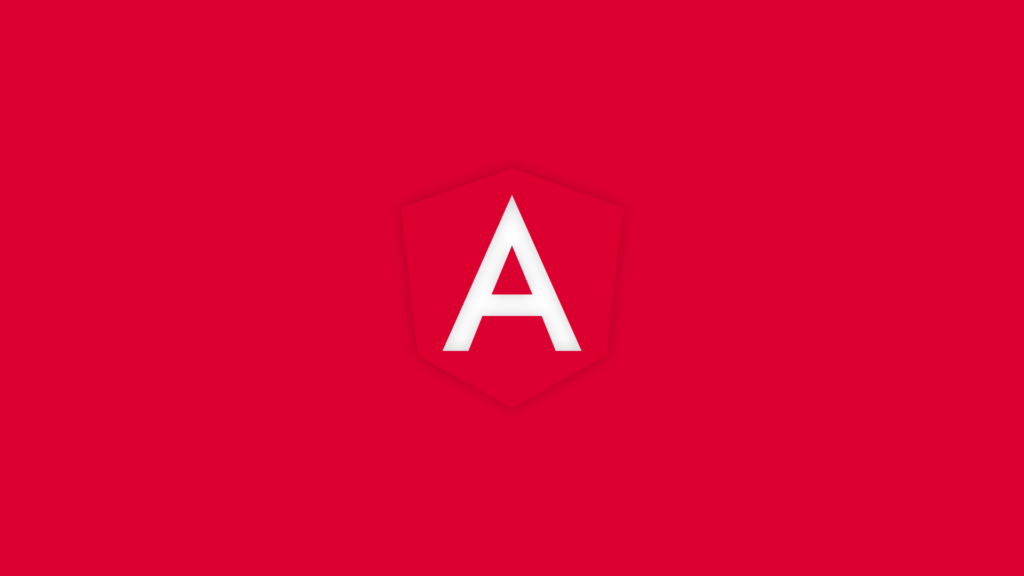
If you’ve built an Angular application before, you’ve probably wondered if there are better ways to accomplish common development tasks. When working, we tend to form habits and continually transfer those habits to new platforms without a second thought. But let’s take a look at some ways to make our development lives easier.
Simplifying Style Paths
Have you ever seen relative path imports in SCSS files associated with your Angular @Component? For example:
@import "../../../theme-variables";
Gets kind of painful with a complex project structure. Even worse when you try to refactor that structure. So what’s the preferred way to import SCSS files? The stylePreprocessorOptions property in your angular.json.
The stylePreprocessorOptions allows you to include additional base paths that Angular will pull in when compiling.
"stylePreprocessorOptions": {
"includePaths": [
"src/theme"
]
}
So now, when your project compiles, imports are simplified into:
@import "theme-variables";
Angular CLI
More than likely, you’ve had some experience with the Angular CLI if you’ve worked with any Angular project. But if you have only heard about it but don’t leverage it, you might want to consider getting familiar with what it offers.
The Angular CLI is a command-line interface that helps initialize, develop, scaffold, and maintain Angular applications. The CLI is a bit more helpful than some of the IDE plugins you may find out there and became even more powerful as of Angular version 6. Let’s take a look at some of the functionality provided by the CLI.
ng generate
Are you still finding yourself creating Angular application classes by hand? With the CLI, there’s a simplified approach using the below command.
ng g component <name>
//ex: ng g component todo
Some things to remember when running these commands.
- Each class that the CLI can generate has its own set of flags. For example, running the above will create an @Component called todo.component.ts and will add it to the root @NgModule. So when creating a new @Component with the CLI, be sure to use the –module flag to specify which Feature Module it should be a part of.
- Generation will create any necessary files. For example, generating an @Component will also create the associated Template, Spec, and SCSS files.
- Run the command in the directory you’d like for the generation to produce its classes in.
ng add
The ng add <package> utilizes your package manager to install dependencies. Those dependencies can have their own installation script which can update your project with configuration changes and additional dependencies. For example, running npm install <package> will install the dependency, whereas the ng add <package> will install the dependency and configure your angular.json
ng update
Given your package.json, the ng update <package> recommends updates to your application. It will help with dependency versioning, and if one of those dependencies provides an update script using schematics, will even update your code.
Feature Modules
Personally, I continually toil over the best structure when building Angular applications. Should this live here?
To help developers organize their code, they introduced the concept of Feature Modules. This best practice helps logically group code into buckets:
- Domain
- Routed
- Routing
- Service
- Widget
Within these groupings, Angular has done a great job of helping guide developers with what should be included in each type of Feature Module. The goal here being a clear separation of concerns for reusable, maintainable, and extensible code.
Build Angular Applications The Easy Way
There are a lot of little tidbits to pick up along the way when building Angular applications. Though their website is a gold mine of documentation, it doesn’t always provide a short list of things to look at. In the future, I hope to provide more in-depth posts for all you Angular fans. Keep checkin’ back or contact us directly to speak to a Rōnin!